Python Syntax Errors: Common Mistakes and How to Fix Them
Flipnode on Jun 15 2023
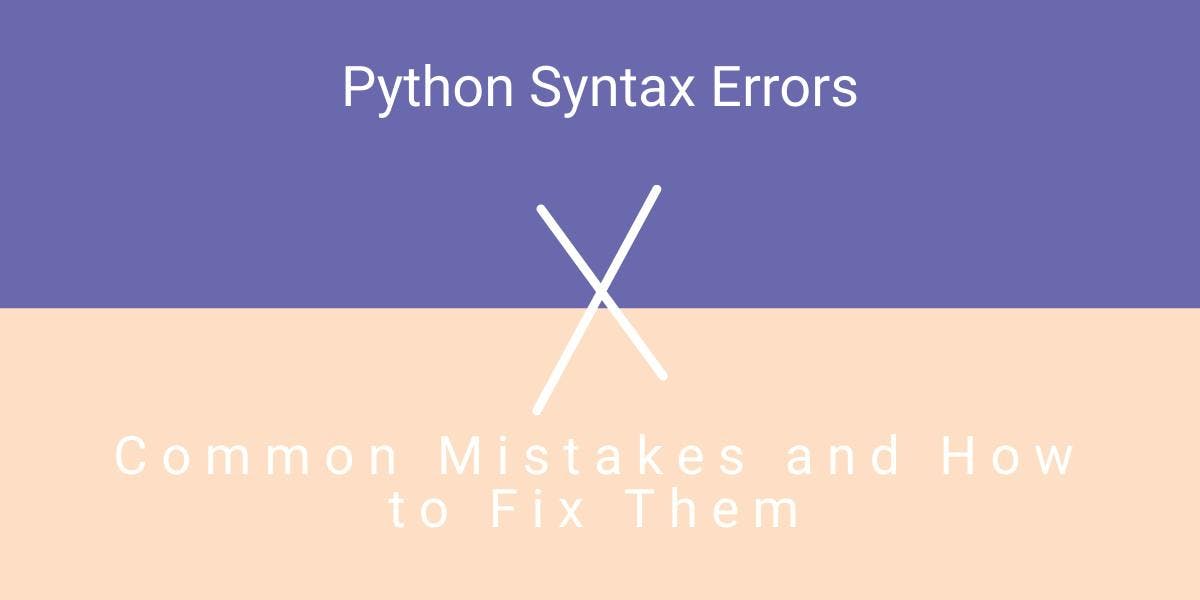
Whether you consider yourself a seasoned Pythonista or you're just starting out with Python programming, you've likely encountered syntax errors along the way. These errors can occur due to something as simple as a missing punctuation mark, and deciphering the error message can sometimes be a challenge.
In this article, we will delve into the world of Python syntax errors, exploring how to interpret them, prevent them, and resolve them effectively. Through practical examples, you'll gain a better understanding of how to navigate and conquer syntax errors in Python.
What are syntax errors in Python?
Syntax errors occur when there are inconsistencies or violations in the structure of the code. In simpler terms, Python syntax errors occur when the Python interpreter cannot comprehend the arrangement of statements in the code.
Like any language, Python follows a set of rules to ensure the intended meaning is conveyed accurately. Omitting certain words or using incorrect syntax can drastically alter the intended message. Programming languages, including Python, adhere to specific syntax rules to ensure code coherence.
Programming languages act as translators, converting human-readable instructions into machine-executable code. Therefore, there are distinct steps involved in the code execution process. When you run your code, the Python interpreter parses it into smaller components, translates them into bytecode, and finally executes it.
During the parsing stage, the interpreter thoroughly examines your code for any invalid syntax. As soon as it encounters an instance of incorrect syntax usage, it promptly notifies you by raising a SyntaxError, an unwelcome message that highlights the issue at hand.
How to read Python syntax errors
When encountering an error message, Python attempts to identify the root cause of the issue. At times, the error message precisely indicates the problem, while in other cases, it can be ambiguous and perplexing. This occurs because Python identifies the first occurrence of syntax it cannot comprehend, potentially displaying an error in a code line that follows the actual error.
Having the ability to interpret Python error messages is immensely beneficial and can save both time and effort. Let's examine a Python web scraping code snippet that triggers two syntax errors:
prices = {"price1": 9.99, "price2": 13.48 "price3": 10.99, "price4": 15.01}
price_found = False
for key value in prices.items():
if 10 <= value <= 14.99:
print(key + ":", value)
price_found = True
if not price_found:
print("There are no prices between $10 and $14.99")
In this example, we have a dictionary containing various prices. We utilize a for loop to find and print the prices between $10 and $14.99. The price_found variable, a boolean value, determines whether such a price exists in the dictionary.
When executed, Python highlights the first encountered invalid syntax error, disregarding two additional errors along the way. The information within the yellow box aids in identifying the error's location, while the green box provides additional details about the error itself. The complete message can be divided into four key elements:
- The file's directory path and name where the error occurred.
- The line number and the specific line of code where the error was initially detected.
- Carets (^) that indicate the precise location of the error.
- The error message, which identifies the type of error and may include supplementary information to resolve the problem.
The code snippet presented a syntax error found in the first line, specifically within the prices dictionary. The carets indicate that the error lies between "price2": 13.48 and "price3": 10.99. The invalid syntax message suggests that we might have forgotten to include a comma between the items in our dictionary. That is indeed the issue! The Python interpreter offers the correct solution, so let's update the code:
prices = {"price1": 9.99, "price2": 13.48, "price3": 10.99, "price4": 15.01}
This time, the carets fail to precisely indicate the error's location, and the SyntaxError message does not provide additional information regarding a potential solution. In such cases, a general guideline is to examine the code immediately preceding the carets. In the code snippet, the syntax error arises due to a missing comma between the variables key and value in the for loop. The correct syntax for the code line should be:
for key, value in prices.items():
Regarding the causes of Python syntax errors, there are instances where the available information might not be sufficient to guide you in the right direction. The example mentioned above illustrates an invalid syntax error without clear details. Therefore, having knowledge of common reasons that lead to syntax errors in Python can greatly assist in resolving them effortlessly.
Common causes of syntax errors
Common Python syntax errors typically arise from the following issues in the code:
- Misplaced, missing, or mismatched punctuation marks such as parentheses, brackets, braces, quotes, commas, colons, etc.
- Misspelled, misplaced, or missing Python keywords.
- Illegal characters used in variable names.
- Incorrect indentation of code blocks.
- Incorrect usage of the assignment operator (=).
These errors often occur due to factors like a lack of familiarity with Python, errors introduced while copy-pasting code, and typographical mistakes. Typing errors, in particular, are easy to make and challenging to identify, so it is recommended to carefully review your code before finalizing it.
Regardless, there are additional measures you can take during coding to reduce the likelihood of encountering syntax errors.
How to avoid Python syntax errors?
To enhance your code and prevent invalid syntax errors in Python, consider following these steps:
- Utilize an integrated development environment (IDE) or a code editor that highlights reserved Python keywords and errors, and provides suggestions while you write code. This initial step is crucial for improving code quality and avoiding syntax errors.
- Test your code frequently as you write it. This proactive approach helps catch syntax errors early on, minimizing the chances of repeating the same mistakes in the future.
- Maintain consistent indentation. Inconsistent indentation is a common cause of syntax errors in Python. Be vigilant in checking your code and utilize IDEs or code editors that highlight indentation errors.
- Employ linting and formatting modules that check your code for errors and style inconsistencies. Some modules can automatically fix code issues, saving you time and effort. Notable examples include pycodestyle, pylint, pyflakes, Flake8, and Black. These tools are especially useful when running Python in the Terminal without an IDE or code editor.
- Refer to the official Python documentation. It contains valuable information that deepens your understanding of Python syntax and provides insights into the meaning of syntax error messages.
Keep in mind that these steps can help you prevent common Python syntax errors, but they do not guarantee complete elimination of such errors.
Additionally, even if your code is syntactically correct, runtime errors can still occur during program execution, altering its normal flow. Unlike syntax errors, runtime errors can be handled using appropriate exception handlers, allowing the program to continue running. Logical errors, on the other hand, occur when code is syntactically valid and runs without errors, but the output does not match the expected result. While this article focuses on invalid syntax issues, it's important to note that SyntaxError exceptions are part of a broader range of exceptions. For advanced Python programming, it is recommended to learn about other types of errors and how to handle them.
How to fix syntax errors
Let's look at some actual code examples and correct them now that we've covered the typical sources of Python syntax problems and general advice on avoiding them.
Misplaced, missing, or mismatched punctuation
1. Ensure Proper Closure of Parentheses, Brackets, and Braces in Python Code
One common cause of syntax errors in Python is the failure to properly close parentheses (), brackets [], and braces {}. When these symbols are left unclosed, the Python interpreter considers everything after the first occurrence as a single statement. Let's take a look at a code sample related to web scraping and fix the issue:
payload = {
"url": "https://www.example.com/",
"filters": {
"crawl": [".*"],
"process": [".*"],
"max_depth": 1,
"scrape_params": {
"user_agent_type": "desktop",
},
"output": {
"type_": "sitemap"
}
}
# Error message
File "<stdin>", line 1
payload = {
^
SyntaxError: '{' was never closed
Although it appears that the payload is enclosed in braces, the interpreter throws a syntax error indicating otherwise. In this specific case, the "filters" parameter lacks the closing brace, which the interpreter does not explicitly mention in its traceback. To fix the error, simply add the missing closing brace for the "filters" parameter:
payload = {
"url": "https://www.example.com/",
"filters": {
"crawl": [".*"],
"process": [".*"],
"max_depth": 1
}, # Closing brace added here
"scrape_params": {
"user_agent_type": "desktop",
},
"output": {
"type_": "sitemap"
}
}
By ensuring the proper closure of parentheses, brackets, and braces, you can avoid syntax errors and ensure the smooth execution of your Python code.
2. Ensure Proper Closure of Strings with Appropriate Quotes
Another common source of syntax errors in Python is the failure to correctly close a string with proper quotes. It is crucial to use matching quotation marks to indicate the beginning and end of a string. Let's explore an example that demonstrates this:
list_of_URLs = (
'https://example.com/1',
'https://example.com/2",
'https://example.com/3
)
print(list_of_URLs)
# Error message
File "<stdin>", line 3
'https://example.com/2",
^
SyntaxError: unterminated string literal (detected at line 3)
In this example, there are two errors, but the interpreter only displays the first syntax error. It points out the exact issue, which is the use of a single quote to start the string and a double quote to close it.
The second error lies in the third URL, which lacks a closing quotation mark altogether. To rectify these errors, ensure that all strings are properly closed with the appropriate quotes:
list_of_URLs = (
'https://example.com/1',
'https://example.com/2',
'https://example.com/3'
)
print(list_of_URLs)
When a string contains quotation marks within its content, use single quotes ('), double quotes ("), or triple quotes (''') to clearly indicate the beginning and end of the string. For example:
print("In this example, there's a 'quote within a quote', which we separate with double and single quotes.")
If you encounter a syntax error, such as the one shown below:
print("In this example, there's a "quote within 'a quote'", which we separate with double and single quotes.")
The interpreter identifies the location of the error, indicated by the caret within the second double quotation mark. To resolve this, you can enclose the entire string within triple quotes (''' or """):
print("""In this example, there's a "quote within 'a quote'", which we specify using different quotes.""")
By carefully closing strings with appropriate quotes, you can avoid syntax errors and ensure the accuracy of your Python code.
3. When passing multiple arguments or values, it is crucial to separate them with commas. Let's consider the following web scraping example that defines HTTP headers in a dictionary:
headers = {
'Accept': 'text/html',
'Accept-Encoding': 'gzip, deflate, br',
'Accept-Language': 'en-US, en;q=0.9'
'Connection': 'keep-alive'
}
# Error message
File "<stdin>", line 5
'Connection': 'keep-alive'
^
SyntaxError: invalid syntax
In this case, a syntax error occurs because there is a missing comma after the 'Accept-Language' argument. To resolve the error, we need to add the missing comma:
headers = {
'Accept': 'text/html',
'Accept-Encoding': 'gzip, deflate, br',
'Accept-Language': 'en-US, en;q=0.9',
'Connection': 'keep-alive'
}
By adding the comma after 'en;q=0.9', the code becomes syntactically correct, and the error is resolved.
4. When defining a function or a compound statement such as if, for, while, or def, it's important to include a colon : at the end. Let's consider the following example of web scraping:
def extract_product_data()
for url in product_urls
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.content, 'html.parser')
title = soup.find("h1").text
price = soup.find("span", {"itemprop": "price"}).text
product_data.append({
"title": title,
"price": price,
})
# Error message
File "<stdin>", line 1
def extract_product_data()
^
SyntaxError: expected ':'
In this case, a syntax error occurs because the def statement and the for loop are missing the required colons. To fix the error, we need to add the missing colons:
def extract_product_data():
for url in product_urls:
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.content, 'html.parser')
title = soup.find("h1").text
price = soup.find("span", {"itemprop": "price"}).text
product_data.append({
"title": title,
"price": price,
})
By adding the colons at the end of the function definition and the for loop, the code becomes syntactically correct, and the error is resolved.
Misspelled, misplaced, or missing Python keywords
1. It's important to avoid using reserved Python keywords as variable or function names. If you're unsure whether a word is a Python keyword or not, you can check it using the keyword module in Python or refer to the reserved keywords list. Many IDEs, such as PyCharm and VS Code, also provide highlighting for reserved keywords, which can be very helpful in identifying them.
In the code snippet below, the reserved keyword pass is used as a variable to hold the password value, resulting in a syntax error:
user = 'username1'
pass = 'password1'
# Error message
File "<stdin>", line 2
pass = 'password1'
^
SyntaxError: invalid syntax
To resolve this error, you need to choose a different name for the variable that doesn't conflict with reserved keywords. Here's an updated version of the code:
user = 'username1'
password = 'password1'
By replacing pass with password, the code becomes syntactically correct, and the error is resolved.
2. It's important to ensure that you haven't misspelled any Python keywords. In the code snippet below, the keyword import is misspelled as impotr when trying to import the Session object from the requests library, resulting in a syntax error:
import time
from requests impotr Session
# Error message
File "<stdin>", line 2
from requests impotr Session
^^^^^^
SyntaxError: invalid syntax
To fix this error, you need to correct the spelling of the keyword import. Here's the corrected code:
import time
from requests import Session
By replacing impotr with import, the code becomes syntactically correct, and the error is resolved.
3. Placing a Python keyword where it shouldn't be will result in an error. It's important to ensure that the keyword is used in the correct syntactical order and follows the rules specific to that keyword. Let's consider the following example:
import time
import Session from requests
# Error message
File "<stdin>", line 2
import Session from requests
^^^^
SyntaxError: invalid syntax
In this case, we encounter an invalid syntax error because the keyword from is used in an incorrect position. To fix this error, we need to rearrange the code to follow the proper syntax. Here's the corrected code:
import time
from requests import Session
By moving the keyword from to the appropriate position, the code becomes syntactically correct, and the error is resolved.
Illegal characters in variable names
Python variables must adhere to certain naming conventions:
- Variable names cannot contain blank spaces. It is recommended to use underscores (_) as a substitute. For example, if you want a variable named "two words", it should be written as two_words, twowords, TwoWords, twoWords, or Twowords.
- Variables in Python are case-sensitive, meaning example1 and Example1 are treated as separate variables. Keep this in mind when creating variables and referring to them later in your code.
- Variable names should not begin with a number. Python will raise a syntax error if you attempt to do so:
response1 = requests.get(url)
2response = requests.post(url)
# Error message
File "<stdin>", line 2
2response = requests.post(url)
^
SyntaxError: invalid decimal literal
As shown in the example, while Python allows numbers to be used within variable names, it does not permit starting a variable name with a number.
4. Variable names can only consist of letters, numbers, and underscores. Using any other characters in the name will result in a syntax error.
Incorrect indentation
1. It is important to remember that certain Python commands, such as compound statements and functions, rely on proper indentation to define their scope. Therefore, make sure that such commands in your code are appropriately indented. Here's an example:
prices = (16.99, 13.68, 24.98, 14.99)
def print_price():
for price in prices:
if price < 15:
print(price)
print_price()
# Error message 1
File "<stdin>", line 6
if price < 15:
^
IndentationError: expected an indented block after 'for' statement on line 5
# Error message 2
File "<stdin>", line 7
print(price)
^
IndentationError: expected an indented block after 'if' statement on line 6
In the above example, the indentation of the if statement and the print statement inside the print_price function is incorrect, resulting in indentation errors. To resolve these errors, ensure that the code within the function is indented properly.
The first error message indicates that the if statement requires an indented block. After fixing that and running the code, we encounter the second error message that tells us the print statement is outside the if statement and requires another indentation. Here's the code with the correct indentation:
prices = (16.99, 13.68, 24.98, 14.99)
def print_price():
for price in prices:
if price < 15:
print(price)
print_price()
By ensuring proper indentation, the code will execute without any indentation errors.
2. To ensure consistent and readable code, it's important to use either spaces or tabs for indentation consistently throughout your codebase. Mixing spaces and tabs can lead to readability issues and may introduce unnecessary syntax errors. Let's fix the first error message in the code sample by using consistent indentation with spaces:
prices = (16.99, 13.68, 24.98, 14.99)
def print_price():
for price in prices:
if price < 15:
print(price)
print_price()
By using spaces for indentation, the code becomes more readable and avoids potential syntax errors. It's a good practice to configure your IDE or code editor to automatically convert tabs to spaces or vice versa based on your preference to maintain consistent indentation throughout your code.
Incorrect use of the assignment operator (=)
1. Ensure that you are not using the assignment operator = to assign values to functions or literals. The assignment operator can only be used to assign values to variables. Let's fix the examples:
Example 1:
price = 10.98
In this case, the string "price" cannot act as a variable, so the quotation marks should be removed.
Example 2:
price = 10.98
print(type(price))
To check whether the value 10.98 is of float type, you can use the type() function and compare it with float using the equality operator ==.
Example 3:
price = 10.98
is_float = type(price) == float
print(is_float)
Here, we assign the result of the comparison type(price) == float to the variable is_float and then print its value.
By using the correct syntax and understanding that the assignment operator should only be used with variables, you can avoid the syntax errors raised by the interpreter.
2. When assigning values in a dictionary, use a colon : instead of the assignment operator =. In the previous code sample, the assignment operator is incorrectly used. Let's fix it:
headers = {
'Accept': 'text/html',
'Accept-Encoding': 'gzip, deflate, br',
'Accept-Language': 'en-US, en;q=0.9',
'Connection': 'keep-alive'
}
By replacing the assignment operators with colons, the dictionary headers is defined correctly without any syntax errors.
3. When comparing objects based on their values, use the double equal sign == instead of the assignment operator =. In the given code sample, the assignment operator is incorrectly used for comparison. Let's fix it:
price_1 = 200.99
price_2 = 200.98
compare = (price_1 == price_2)
print(compare)
By using == between price_1 and price_2, the code will compare the values and store the result in the compare variable. The correct comparison will be performed, and the result will be printed accordingly.
Final thoughts
Understanding and debugging syntax errors in Python can be challenging, but with the knowledge of error tracebacks, common causes, and their solutions, you can minimize the time and effort spent on debugging. This article has provided you with insights to help you produce Python code that is free of syntax errors. Armed with this information, you are well-equipped to write code that is syntactically flawless.