Python Cache: How to Speed Up Your Code with Effective Caching
Flipnode on May 26 2023
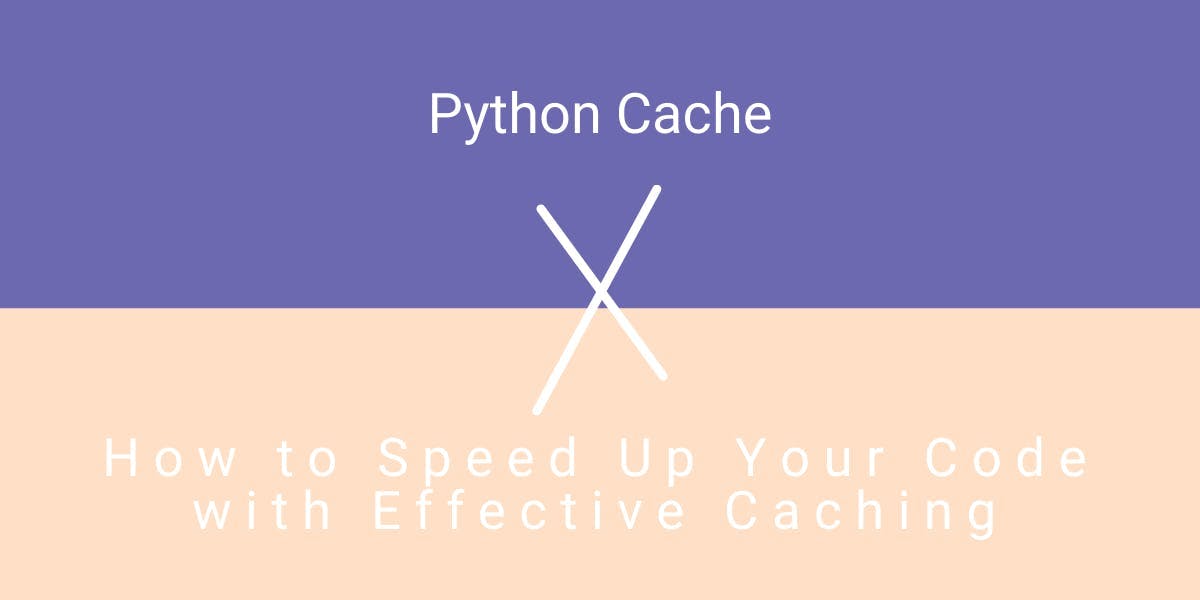
A smooth and seamless user experience is vital for the success of any user-facing application. Developers are constantly aiming to minimize application latencies to enhance the user experience, with data access delays often being the primary culprit.
One effective way to reduce these delays is through data caching, which results in faster load times and increased user satisfaction. Web scraping projects can also benefit significantly from caching, enabling substantial speed improvements.
So, what is caching, and how can it be implemented? This article will delve into the concept of caching, its purpose, practical applications, and provide guidance on leveraging caching to optimize your web scraping code using Python.
What is a cache in programming?
Caching serves as a mechanism to enhance the performance of applications. Essentially, caching involves storing data in a designated cache and retrieving it later when needed. But what exactly is a cache?
A cache is a rapid storage space, typically temporary, where frequently accessed data is stored to accelerate system performance and reduce access times. For instance, in a computer, the cache is a small but swift memory chip, often SRAM, positioned between the CPU and the main memory chip, commonly DRAM.
When the CPU requires data, it initially checks the cache. If the data is present in the cache, a cache hit occurs, enabling the CPU to retrieve the data from the cache instead of the relatively slower main memory. This leads to reduced access times and improved overall performance.
The purpose of caching
Caching offers multiple benefits for applications and systems, contributing to their enhanced performance in various ways. Here are the primary advantages of utilizing caching:
- Accelerated access time:
Caching focuses on expediting the retrieval of frequently accessed data. By storing such data in a readily accessible temporary storage area, caching reduces the time required to retrieve it from the original data source. This significant decrease in access time significantly improves the overall performance of applications and systems. - Reduced system load:
Caching effectively alleviates the burden on the system by minimizing the number of requests made to the external data source, such as a database. By storing frequently used data in the cache, applications can retrieve the data from the cache instead of repeatedly querying the data source. Consequently, this reduces the workload on the external data source, leading to improved system performance. - Enhanced user experience:
Caching plays a crucial role in providing users with rapid access to data, enabling smoother interaction with the system or application. This aspect is particularly critical for real-time systems and web applications where users expect instantaneous responses. By facilitating quicker data retrieval, caching significantly contributes to improving the overall user experience of an application or system.
Common use cases for caching
Caching is a versatile concept with several notable applications across various domains. It can be implemented in scenarios where data access follows predictable patterns, allowing for proactive caching of anticipated data and improving application performance.
Here are some prominent use cases where caching can be applied:
- Web applications:
Caching plays a crucial role in web applications that frequently retrieve data from databases or external APIs. By caching frequently accessed data, such as database query results or API responses, the access time can be significantly reduced, leading to improved overall performance. Caching can be utilized both on the client side, where static resources and user preferences are stored in the browser cache, and on the server side, where in-memory caching between the web servers and databases can reduce the request load on the database, resulting in faster load times. For example, in an e-commerce website, caching the list of products using in-memory caching can prevent repeated database access and enhance user experience when browsing multiple products. - Machine learning:
Caching is beneficial in machine learning applications that involve large datasets. By prefetching subsets of the dataset into the cache, the data access time is reduced, resulting in faster model training. Additionally, caching weight vectors of trained machine learning models enables quick access for predicting new unseen samples, which is a common operation in many applications. - Central processing units (CPUs):
CPUs employ dedicated caches (such as L1, L2, and L3 caches) to optimize their operations. These caches prefetch data based on spatial and temporal access patterns, saving valuable CPU cycles that would otherwise be wasted on reading from the main memory (RAM). The use of CPU caches significantly improves performance by minimizing data access latency.
In each of these use cases, caching proves to be a valuable technique, enhancing performance by proactively storing and retrieving frequently accessed data, resulting in faster data access times and overall improved system efficiency.
Caching Strategies
Different caching strategies can be developed based on specific spatial or temporal data access patterns.
Spatial caching is commonly used in scientific or technical applications that handle large volumes of data and require high performance. It involves prefetching data that is spatially close (in memory) to the currently processed data. This strategy takes advantage of the likelihood that the program or application user will demand data units that are spatially adjacent to the current demand. Prefetching spatially close data can save time and improve performance.
Temporal caching, on the other hand, is a more popular strategy and focuses on retaining data in the cache based on its frequency of use. Several popular temporal caching strategies exist:
- First-In, First-Out (FIFO):
This approach operates on the principle that the first item added to the cache will be the first one to be removed. A predetermined number of items is loaded into the cache, and when the cache reaches its capacity, the oldest item is evicted to accommodate a new one. FIFO is suitable for systems prioritizing access order, such as message processing or queue management systems. - Last-In, First-Out (LIFO):
LIFO caching replaces the most recently added item in the cache first. As new items are added, the most recent addition is the first one to be removed to make space for the newest item, while the oldest item remains in the cache until eviction. This strategy is useful for applications that prioritize recent data over older data, such as stack-based data structures or real-time streaming. - Least Recently Used (LRU) Caching:
LRU caching involves storing frequently used items in the cache and evicting the least recently used items when space is needed. This strategy is commonly used in web applications or database systems, where frequently accessed data is more important than older data. In LRU caching, the item that hasn't been used recently is evicted first. - Most Recently Used (MRU) Caching:
MRU caching evicts items based on their most recent use. Unlike LRU caching, which removes the least recently used items first, MRU caching removes the items that have been accessed most recently. This strategy is beneficial in scenarios where items that were recently used are more likely to be used again. - Least Frequently Used (LFU) Caching:
LFU caching removes the item that has been accessed the least number of times since its addition to the cache. LFU doesn't rely on storing access times but instead keeps track of the number of times an item has been accessed since it was added. This strategy is helpful when the frequency of use is an important factor in determining cache eviction.
By employing these caching strategies, applications and systems can optimize their data access patterns and improve overall performance by efficiently managing the cache and maximizing the utilization of frequently accessed data.
How to implement a cache in Python
In Python, there are various methods available to implement caching for different caching strategies. In this example, we will explore two methods of Python caching specifically for a simple web scraping scenario.
To begin, you'll need to install the necessary libraries. We will be using the requests library for making HTTP requests to a website. You can install it using pip with the following command in your terminal:
python -m pip install requests
For this project, we will also utilize the time and functools libraries, which are already included with Python 3.11.2, so there is no need to install them separately.
Method 1: Python caching using a manual decorator
In Python, decorators are functions that take another function as input and return a new function, allowing us to modify the behavior of the original function without altering its source code.
One common use case for decorators is implementing caching. This involves creating a dictionary to store the results of a function and retrieving them from the cache for subsequent calls.
Let's begin by defining a simple function that takes a URL as an argument, sends a request to that URL, and returns the response text:
import requests
def get_html_data(url):
response = requests.get(url)
return response.text
Now, we'll create a memoized version of this function using a decorator:
def memoize(func):
cache = {}
def wrapper(*args):
if args in cache:
return cache[args]
else:
result = func(*args)
cache[args] = result
return result
return wrapper
@memoize
def get_html_data_cached(url):
response = requests.get(url)
return response.text
The memoize function is a decorator that generates a cache dictionary to store the results of previous function calls. The wrapper function checks if the current input arguments are already in the cache and returns the cached result if available. Otherwise, it calls the original function, stores the result in the cache, and returns the result.
By applying the @memoize decorator above the get_html_data function, we create a new memoized function called get_html_data_cached. This function only makes a single network request for a URL and stores the response in the cache for subsequent requests.
To compare the execution speeds of the original function and the memoized function, we can use the time module:
import time
start_time = time.time()
get_html_data('https://books.toscrape.com/')
print('Time taken (normal function):', time.time() - start_time)
start_time = time.time()
get_html_data_cached('https://books.toscrape.com/')
print('Time taken (memoized function using manual decorator):', time.time() - start_time)
This code snippet measures the time taken for the get_html_data function and the get_html_data_cached function to execute. By comparing the times, we can observe the difference in performance.
Here's the complete code:
import time
import requests
def get_html_data(url):
response = requests.get(url)
return response.text
def memoize(func):
cache = {}
def wrapper(*args):
if args in cache:
return cache[args]
else:
result = func(*args)
cache[args] = result
return result
return wrapper
@memoize
def get_html_data_cached(url):
response = requests.get(url)
return response.text
start_time = time.time()
get_html_data('https://books.toscrape.com/')
print('Time taken (normal function):', time.time() - start_time)
start_time = time.time()
get_html_data_cached('https://books.toscrape.com/')
print('Time taken (memoized function using manual decorator):', time.time() - start_time)
By comparing the execution times, you can see the difference in performance between the normal function and the memoized function.
Output:
Time taken (normal function): 0.682342529296875
Time taken (memoized function using manual decorator): 0.6632871627807617
Note: Since we are making only one request in this example, the memoized function doesn't show a significant time difference compared to the normal
Method 2: Python caching using LRU cache decorator
Another method for implementing caching in Python is by using the built-in @lru_cache decorator from the functools module. This decorator utilizes the least recently used (LRU) caching strategy, where data that hasn't been used recently will be discarded from the cache.
To use the @lru_cache decorator, we need to create a new function for retrieving HTML content and place the decorator above it. Make sure to import the functools module before using the decorator:
from functools import lru_cache
@lru_cache(maxsize=None)
def get_html_data_lru(url):
response = requests.get(url)
return response.text
In the above example, the get_html_data_lru function is memoized using the @lru_cache decorator. By setting the maxsize option to None, the cache can grow indefinitely.
To utilize the @lru_cache decorator, simply add it above the get_html_data_lru function. Here's the complete code sample:
from functools import lru_cache
import time
import requests
def get_html_data(url):
response = requests.get(url)
return response.text
@lru_cache(maxsize=None)
def get_html_data_lru(url):
response = requests.get(url)
return response.text
start_time = time.time()
get_html_data('https://books.toscrape.com/')
print('Time taken (normal function):', time.time() - start_time)
start_time = time.time()
get_html_data_lru('https://books.toscrape.com/')
print('Time taken (memoized function with LRU cache):', time.time() - start_time)
This code measures the execution times of the normal function and the memoized function with the LRU cache.
Output:
Time taken (normal function): 0.682342529296875
Time taken (memoized function with LRU cache): 0.09025692939758301
As shown in the output, the memoized function using the LRU cache demonstrates a significant improvement in execution time compared to the normal function.
Conclusion
Caching data can be a valuable technique to optimize your code and enhance its speed. In various scenarios, caching proves to be a game changer, and web scraping is no exception. Particularly for large-scale projects, incorporating caching for frequently accessed data can significantly accelerate data extraction processes and enhance overall performance.