HTTPX vs Requests vs AIOHTTP
Flipnode on Jun 08 2023
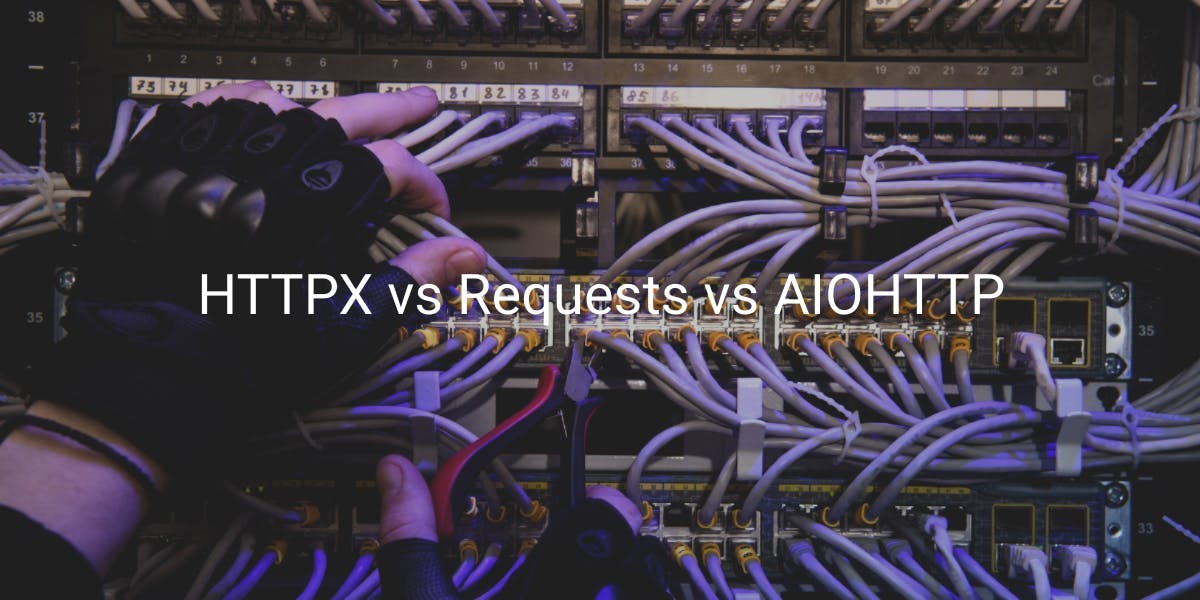
HTTPX is a Python library designed to enhance the experience of making HTTP requests by providing modern features and improved capabilities. It was specifically developed to support asynchronous programming, which has contributed to its growing popularity within the Python community. This article aims to compare HTTPX with two other well-known Python HTTP clients, namely Requests and AIOHTTP, considering their feature-rich APIs and performance.
How HTTPX compares to the Requests module
Requests is a widely-used Python library renowned for its simplicity and popularity when it comes to making HTTP requests. It has been the preferred choice for many developers due to its straightforward API and long-standing presence. If you want to delve deeper into Requests, we recommend referring to our comprehensive guide on the Python Requests Library.
In comparison, HTTPX is a relatively newer HTTP client for Python that offers a more extensive range of features compared to the widely-used Requests library. Here are some notable distinctions between HTTPX and Requests:
- Async compatibility: HTTPX supports both asynchronous and synchronous programming, making it suitable for both types of applications. In contrast, the Requests library is primarily synchronous and lacks built-in support for asynchronous operations.
- HTTP/2 support: HTTPX supports the latest HTTP protocol version, HTTP/2, which delivers improved performance and more efficient transport. In contrast, Requests does not include native support for HTTP/2.
- Automatic decoding: HTTPX incorporates automatic decoding of JSON and other common formats, making it convenient to handle structured data in responses. This feature can save developers time and effort. Requests, however, requires manual handling of decoding JSON data.
- Size considerations: HTTPX is slightly larger in size compared to Requests. If file size is a concern for your application, this difference may be worth considering.
- Performance: In most cases, HTTPX exhibits better performance than Requests.
Here are some syntax examples showcasing the differences between the two libraries. The following code demonstrates a GET request using the Requests library:
import requests
response = requests.get("https://example.com")
print(response.text)
The equivalent code using the HTTPX library is as follows:
import httpx
response = httpx.get("https://example.com")
print(response.text)
Alternatively, you can create an HTTPX client object and utilize its get method:
client = httpx.Client()
response = httpx.get("https://example.com")
For a POST request with the Requests library, use the post method:
response = requests.post(url, data={"name": "John", "age": 30})
The equivalent syntax using HTTPX is quite similar:
client = httpx.Client()
response = client.post("https://httpbin.org/post", data={"name": "John", "age": 30})
Both libraries share similar usage patterns in simple synchronous scenarios. However, the notable distinction is that HTTPX allows you to write asynchronous code using Python's async/await syntax. Here's an example:
import httpx
import asyncio
async def main():
async with httpx.AsyncClient() as client:
response = await client.get("https://example.com")
print(response.text)
asyncio.run(main())
Though the syntax for making requests with HTTPX is slightly more verbose due to the async/await syntax, it grants you the ability to execute asynchronous HTTP requests, which can be invaluable in certain scenarios.
Additionally, HTTPX offers automatic decoding of JSON and other common formats, which simplifies working with structured data in responses. Consider the following example:
import httpx
response = httpx.get("http://httpbin.org/get")
data = response.json()
print(data["headers"]["User-Agent"])
In this case, the HTTPX library automatically decodes the JSON response, allowing easy access to the headers.
Furthermore, HTTPX includes native support for the newer HTTP protocol version, HTTP/2. To leverage HTTP/2 with HTTPX, install the optional HTTP/2 component using pip:
$ pip install 'httpx[http2]'
Next, when creating an instance of the client, set the optional http2 flag to True:
client = httpx.Client(http2=True)
response = client.get("https://example.com")
print(response.http_version) # Prints HTTP/2
The provided code snippet demonstrates how to determine the HTTP protocol version used, with HTTP/2 being the case in this example. It's important to note that while HTTPX offers HTTP/2 support, Requests does not include this feature.
For more detailed information on HTTP/2, we recommend referring to the provided comprehensive document.
Overall, HTTPX presents a compelling alternative to Requests, incorporating advanced features, improved performance, async compatibility, and automatic decoding capabilities.
Features that make HTTPX stand out
HTTPX offers a range of compelling features that make it a robust and versatile HTTP client for Python. These features include support for async/await syntax, compatibility with synchronous programming, the HTTP/2 protocol, and the ability to handle streaming responses.
One notable feature of HTTPX is its built-in support for streaming responses, which is particularly useful when dealing with large amounts of data. Streaming responses allow you to avoid loading the entire response body into memory at once, providing a more memory-efficient approach.
It's important to mention that AIOHTTP, another popular library, also offers a wide range of valuable options and features that can be beneficial in various scenarios.
An overview of AIOHTTP
AIOHTTP is a client for Python that is designed for use in asynchronous programs. Similarly to HTTPX, it’s built on top of the asyncio library and supports async/await syntax meaning AIOHTTP is a good choice for developers who are already familiar with asyncio and want a lightweight library for making HTTP requests. To find out more, visit the official website.
Like HTTPX, AIOHTTP supports standard HTTP methods. It also includes support for cookies, redirects, and custom headers. AIOHTTP is particularly well-suited for making HTTP requests in high-concurrency environments due to its async-first design and efficient use of resources.
Here is an example of making an asynchronous GET request using AIOHTTP:
import aiohttp
import asyncio
async def main():
async with aiohttp.ClientSession() as session:
async with session.get("https://example.com") as response:
print(await response.text())
asyncio.run(main())
AIOHTTP also supports making asynchronous POST requests and other HTTP methods similarly. Here is an example of making a POST request with AIOHTTP:
import aiohttp
import asyncio
async def main():
async with aiohttp.ClientSession() as session:
async with session.post("http://httpbin.org/post", data={"key": "value"}) as response:
print(await response.text())
asyncio.run(main())
HTTPX vs AIOHTTP
When comparing HTTPX and AIOHTTP, there are several notable differences in terms of library scope and programming approach. HTTPX is a comprehensive HTTP client that caters to a wide range of applications, offering extensive features and capabilities. On the other hand, AIOHTTP focuses on providing a streamlined and efficient API primarily for making HTTP requests in asynchronous programs.
One significant distinction is that HTTPX supports both synchronous and asynchronous programming paradigms, whereas AIOHTTP is exclusively designed for asynchronous programming. Additionally, AIOHTTP goes beyond sending HTTP requests and includes the ability to create HTTP servers.
In most cases, HTTPX can be employed in a broader array of projects, while AIOHTTP is particularly suitable for async-only projects, offering optimization for async programming.
Overall, HTTPX and AIOHTTP are powerful and flexible HTTP clients for Python, each with its own set of features and advantages tailored to different project requirements. However, there are a few key differences worth considering:
- Async compatibility: HTTPX supports both synchronous and asynchronous programming, while AIOHTTP is exclusively asynchronous.
- HTTP/2 support: HTTPX includes support for the latest HTTP protocol version, HTTP/2, whereas AIOHTTP lacks this feature.
- Performance: AIOHTTP is widely recognized for its excellent performance compared to HTTPX. For a more detailed analysis, refer to the next section.
Ultimately, the choice between HTTPX and AIOHTTP depends on your specific needs and the nature of your project.
Performance Comparison of AIOHTTP and HTTPX
To evaluate the performance of AIOHTTP and HTTPX, we can construct a simple program that sends multiple asynchronous GET requests to a website. Here's an example of such a program:
import asyncio
import time
import httpx
import aiohttp
async def main():
# Create clients for both libraries
httpx_client = httpx.AsyncClient()
aiohttp_client = aiohttp.ClientSession()
try:
# Send 100 asynchronous GET requests using HTTPX
start_time = time.perf_counter()
tasks = [httpx_client.get("https://example.com") for _ in range(100)]
await asyncio.gather(*tasks)
end_time = time.perf_counter()
print(f"HTTPX: {end_time - start_time:.2f} seconds")
# Send 100 asynchronous GET requests using AIOHTTP
start_time = time.perf_counter()
tasks = [aiohttp_client.get("https://example.com") for _ in range(100)]
await asyncio.gather(*tasks)
end_time = time.perf_counter()
print(f"AIOHTTP: {end_time - start_time:.2f} seconds")
finally:
# Close client sessions
await aiohttp_client.close()
await httpx_client.aclose()
asyncio.run(main())
Here are the results obtained by running this code on a machine with an M1 processor and gigabit internet connection:
HTTPX: 1.22 seconds
AIOHTTP: 1.19 seconds
When we increase the number of requests to 1000, the results change significantly:
HTTPX: 10.22 seconds
AIOHTTP: 3.79 seconds
From these results, it is evident that AIOHTTP outperforms HTTPX in this particular scenario. However, it's crucial to note that the performance difference between the two libraries may vary depending on the specific use case and the underlying hardware.
It's advisable to conduct your own performance evaluations based on your unique requirements and system configurations to determine which library is the most suitable for your project.
Conclusion
Ultimately, selecting an HTTP client library depends on the specific requirements of your project. If simplicity and ease of use are paramount, Requests may be the ideal choice. On the other hand, if you require a library with advanced features and asynchronous support, HTTPX might be a better fit.
However, it's important to note that HTTPX and AIOHTTP are not the sole options for making HTTP requests in Python. There are numerous other libraries available, each offering its own set of features and trade-offs. Some notable alternatives include http.client, urllib3, and httplib2, among others.
It's recommended to assess the specific needs and constraints of your project and explore the available options to determine the most suitable HTTP client library for your use case.