Using Python and Beautiful Soup to Parse Data: Intro Tutorial
Flipnode on May 31 2023
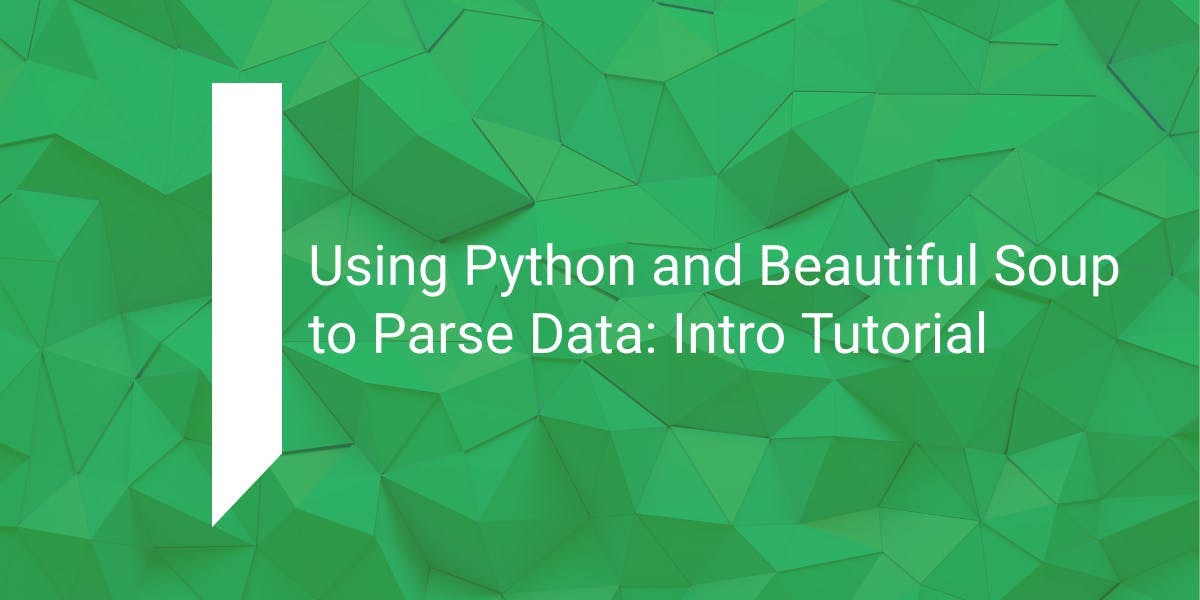
Web scraping can be a complex field, but thanks to coding languages like Python, building a basic web scraper is relatively straightforward. Python offers a range of useful libraries that simplify the process, and one such library is Beautiful Soup. Beautiful Soup is a popular Python package used for parsing HTML and XML documents, and in this tutorial, we will focus on using it to parse data in Python.
While we recommend checking out our comprehensive article or video tutorial on Python web scraping for beginners, this tutorial specifically concentrates on parsing data using a sample HTML file. It aims to provide a quick introduction to the value offered by Python and Beautiful Soup v4. By following the examples provided, you will gain an understanding of the fundamental principles of parsing HTML data. The examples cover traversing a document for HTML tags, printing the content of tags, finding elements by ID, extracting text from specific tags, and exporting it to a .csv file.
Before diving into the main topic, let's review some fundamental concepts.
What is data parsing?
Data parsing refers to the process of analyzing and extracting specific information from a structured or semi-structured data source. It involves breaking down the data into smaller components or fields and interpreting its structure according to predefined rules or patterns.
Parsing is commonly used in various fields, including programming, data analysis, and data integration. In the context of programming, data parsing often refers to the extraction and manipulation of data from formats such as XML, JSON, HTML, CSV, or plain text. It involves identifying the data elements, their relationships, and their corresponding values.
During the parsing process, the data is typically converted into a more usable or structured format that can be further processed or analyzed. This may involve transforming the data into a specific data model, such as a dictionary, list, or object, to facilitate easier access and manipulation.
Overall, data parsing is a crucial step in working with data, as it enables the extraction of relevant information and facilitates further analysis, visualization, or integration with other systems.
What is Beautiful Soup?
Beautiful Soup is a Python library designed for parsing HTML and XML documents. It generates a hierarchical parse tree based on predefined criteria, allowing users to easily extract, navigate, search, and manipulate data from HTML. Beautiful Soup is particularly useful for web scraping tasks. It supports both Python 2.7 and Python 3, making it accessible to a wide range of programmers. With its convenient features, Beautiful Soup can significantly reduce development time for various projects.
Installing Beautiful Soup
Before proceeding with this tutorial, it's essential to have a Python programming environment set up on your machine. For the purpose of this tutorial, we'll assume you're using PyCharm as it provides a convenient option, even for those who are less experienced with Python. However, you can use any IDE of your choice.
If you're using Windows, ensure that you select the "PATH installation" checkbox while installing Python. This will add the Python executables to the default Windows Command Prompt search, allowing you to use commands like "pip" or "python" without specifying the executable's directory. This simplifies the process and makes it more convenient.
Additionally, you need to have Beautiful Soup installed on your system. Regardless of the operating system, you can easily install the latest version of Beautiful Soup by executing the following command in the terminal:
pip install BeautifulSoup4
If you're using Windows, it's recommended to run the terminal as an administrator to ensure a smooth installation process.
Lastly, since we'll be working with a sample HTML file, it's beneficial to have some familiarity with the structure of HTML.
Getting started
To demonstrate the main methods of how Beautiful Soup parses data, we will use a sample HTML file. Although this file is simpler than a typical modern website, it will suffice for the purposes of this tutorial.
Here is the content of the HTML file:
html
Copy code
<!DOCTYPE html>
<html>
<head>
<title>What is a Proxy?</title>
<meta charset="utf-8">
</head>
<body>
<h2>Proxy types</h2>
<p>
There are many different ways to categorize proxies. However, two of the most popular types are residential and data center proxies. Here is a list of the most common types.
</p>
<ul id="proxytypes">
<li>Residential proxies</li>
<li>Datacenter proxies</li>
<li>Shared proxies</li>
<li>Semi-dedicated proxies</li>
<li>Private proxies</li>
</ul>
</body>
</html>
To use this file in PyCharm, simply copy the contents and save it with the .html extension in the directory of your PyCharm project.
Next, open PyCharm and right-click in the project area. Navigate to "New" -> "Python File". Congratulations! You now have a new playground to work with.
Traversing for HTML tags
To extract a list of all the tags used in our sample HTML file using Beautiful Soup, we can follow these steps:
from bs4 import BeautifulSoup
with open('index.html', 'r') as f:
contents = f.read()
soup = BeautifulSoup(contents, features="html.parser")
for child in soup.descendants:
if child.name:
print(child.name)
After running this code, you should see the following output:
html
head
title
meta
body
h2
p
ul
li
li
li
li
li
Now, let's take a closer look at what each line of the code does:
from bs4 import BeautifulSoup
This line imports the Beautiful Soup library.
with open('index.html', 'r') as f:
contents = f.read()
Here, we open the sample HTML file and read its contents.
soup = BeautifulSoup(contents, features="html.parser")
This line creates a BeautifulSoup object and uses Python's built-in HTML parser to parse the HTML contents.
for child in soup.descendants:
if child.name:
print(child.name)
The loop iterates over the descendants of the HTML file and checks if each element has a name (i.e., it is an HTML tag). If it does, the tag name is printed.
This code demonstrates how Beautiful Soup can traverse an HTML file and extract HTML tags. Later in the tutorial, we will explore additional functionalities, such as exporting the results to a .csv file.
Getting the full content of tags
from bs4 import BeautifulSoup
with open('index.html', 'r') as f:
contents = f.read()
soup = BeautifulSoup(contents, features="html.parser")
print(soup.h2)
print(soup.p)
print(soup.li)
This code will output the HTML tags along with their full content in the specified order. The output should look like this:
<h2>Proxy types</h2>
<p>
There are many different ways to categorize proxies. However, two of the most popular types are residential and data center proxies. Here is a list of the most common types.
</p>
<li>Residential proxies</li>
If you want to remove the HTML tags and print only the text, you can use the text attribute of the tag, like this:
print(soup.li.text)
In our case, it will give the following output:
Residential proxies
Keep in mind that these methods only print the first instance of the specified tag. To find elements by ID or filter elements using specific criteria, we can use the find_all method, which we will explore in the following sections.
Using Beautiful Soup to find elements by ID
To find elements by ID in Beautiful Soup, there are two similar ways you can use:
print(soup.find('ul', attrs={'id': 'proxytypes'}))
or
print(soup.find('ul', id='proxytypes'))
Both of these methods will output the same result in the Python Console:
<ul id="proxytypes">
<li>Residential proxies</li>
<li>Datacenter proxies</li>
<li>Shared proxies</li>
<li>Semi-dedicated proxies</li>
<li>Private proxies</li>
</ul>
These methods allow you to locate elements by their ID attribute and retrieve the corresponding HTML code.
Finding all specified tags and extracting text
The find_all method in Beautiful Soup is a powerful tool for extracting specific data from an HTML file. It allows you to filter data based on various criteria. However, for this tutorial, we'll keep it simple and use it to find all items in our list and print their text content only:
from bs4 import BeautifulSoup
with open('index.html', 'r') as f:
contents = f.read()
soup = BeautifulSoup(contents, features="html.parser")
for tag in soup.find_all('li'):
print(tag.text)
Here's the output you should see:
Residential proxies
Datacenter proxies
Shared proxies
Semi-dedicated proxies
Private proxies
Congratulations! You now have a basic understanding of how Beautiful Soup can be used to parse data. It's important to note that the examples in this tutorial serve as introductory material, and real-world web scraping and data parsing scenarios can be more complex. For a more in-depth exploration of Beautiful Soup, I recommend referring to its documentation, which is an excellent resource to further enhance your knowledge.
Exporting data to a .csv file
A practical application of Beautiful Soup is exporting data to a .csv file for further analysis. Although this topic is beyond the scope of this tutorial, let's briefly explore how it can be accomplished.
First, you'll need to install the pandas library, which helps in creating structured data in Python. You can easily install it using the following command:
pip install pandas
Next, add the following line at the beginning of your code to import the library:
import pandas as pd
Moving forward, let's add some lines of code that export the list we extracted earlier to a .csv file. Here's the updated code:
from bs4 import BeautifulSoup
import pandas as pd
with open('index.html', 'r') as f:
contents = f.read()
soup = BeautifulSoup(contents, features="html.parser")
results = soup.find_all('li')
df = pd.DataFrame({'Names': results})
df.to_csv('names.csv', index=False, encoding='utf-8')
What happened here? Let's break it down:
results = soup.find_all('li')
This line finds all instances of the <li> tag and stores them in the results object.
df = pd.DataFrame({'Names': results})
df.to_csv('names.csv', index=False, encoding='utf-8')
In these lines, the pandas library comes into play. It stores our results in a table-like structure called a DataFrame and exports it to a .csv file.
If everything went well, a new file named names.csv should appear in your Python project directory. Inside the file, you should see a table with the list of proxy types. Congratulations! You now not only understand how to extract data from an HTML file but also how to programmatically export it to a new file.
Conclusion
Beautiful Soup is an incredibly useful HTML parser that offers a wide range of capabilities. It has a relatively gentle learning curve, allowing you to quickly understand how to navigate, search, and modify the parse tree. Additionally, when combined with libraries like pandas, you gain even more power to manipulate and analyze the data. This combination opens up endless possibilities for collecting and analyzing data in various use cases. Beautiful Soup, along with its integration with other libraries, provides a powerful package for data collection and analysis.